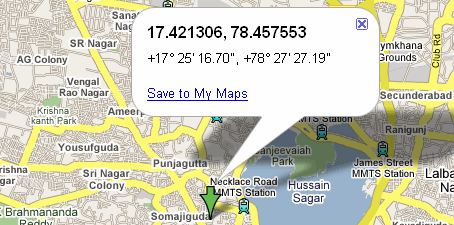
Get latitude and longitude based on location name google maps api
how to get Latitude and Longitude in Google Maps using PHP
The idea is simple. Make a request to Google Maps server, and it will return an XML (or JSON). Then, parse the XML to get the Lat and Lon.
- function Get_Lat_lng($address=null)
- {
- if(!emptyempty($address)){
- $prepAddr = str_replace(‘ ‘,‘+’,$address);
- $geocode=file_get_contents(‘http://maps.google.com/maps/api/geocode/json?address=’.$prepAddr.‘&sensor=false’);
- $output= json_decode($geocode, true);
- if($output[‘status’] ==‘OK’)
- {
- $lat = $output[‘results’][0][‘geometry’][‘location’][‘lat’];
- $lng = $output[‘results’][0][‘geometry’][‘location’][‘lng’];
- $map_code=array(‘status’=>‘ok’,‘lat’=>$lat,‘lng’=>$lng);
- return $map_code;
- }
- else{
- $map_code=array(‘status’=>‘zero’,‘lat’=>‘00.00’,‘lng’=>‘00.00’);
- return $map_code;
- }
- }else{
- $map_code=array(‘status’=>‘zero’,‘lat’=>‘00.00’,‘lng’=>‘00.00’);
- return $map_code;
- }
- }
Now just call Get_Lat_lng($address) and you get latitude and longitude in array
for eg. $address= ” 1600 Amphitheatre Parkway Mountain View, CA 94043 USA”; (google usa office address)
Array ( [status] => ok [lat] => 37.4221913 [lng] => -122.0845853 )